以下のページに、実際に検索をして結果を確認する環境を作りました。
デモ画面サンプル
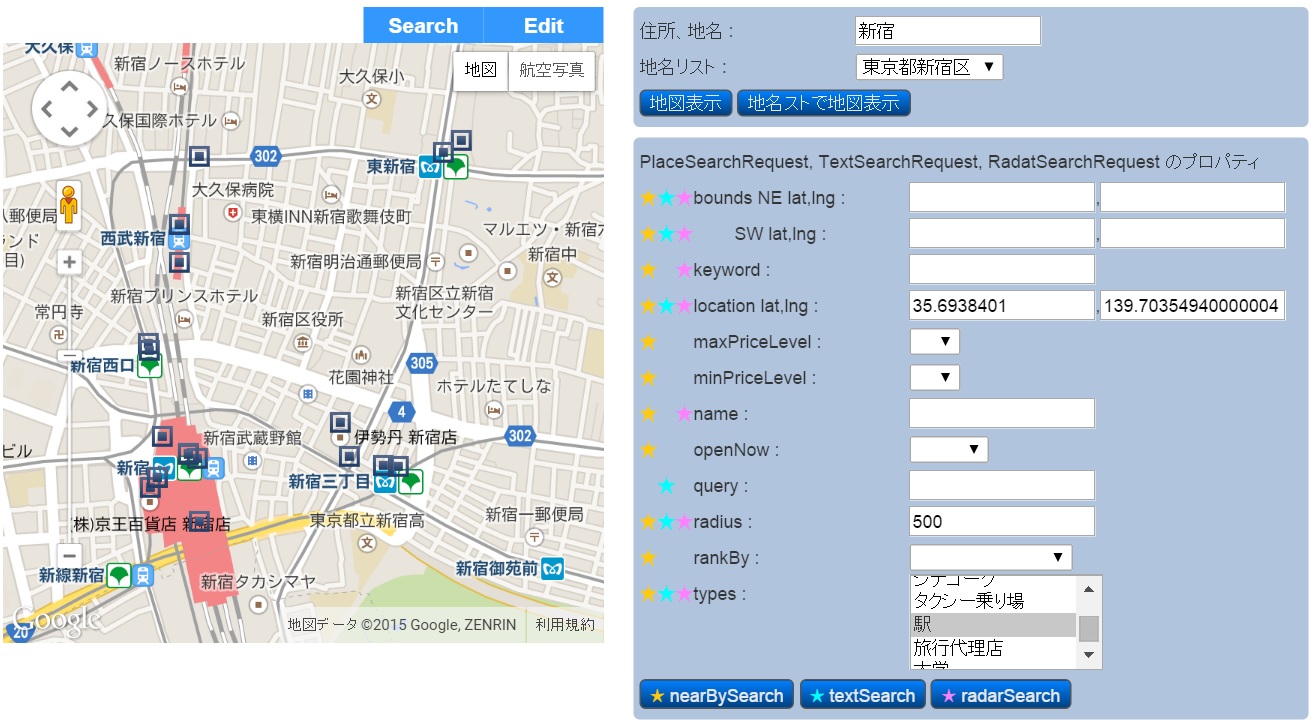
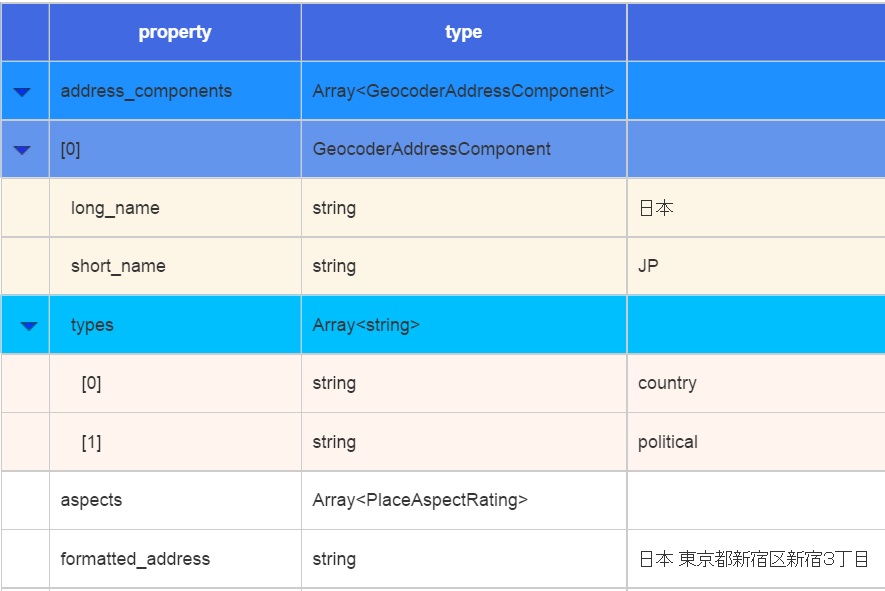
実際に操作してみてください。
以下の表は、この検索操作をして、調べました。
Google Maps JavaScript API v3 の導入は、google のデベロッパーガイドを参照してください。
サンプルのコーディング例もあります。
PlaceResultの構造
PlaceResult は以下のような構造となっています。getDetails 呼び出しでは、登録してあれば、すべての項目が格納されるようです。
PlaceResult
┃
┃
┣━GeocoderAddressComponentの配列 住所を要素に分割
┃
┃
┃
┣━PlaceAspectRatingの配列
┃
┃
┃
┣━PlaceGeometry 緯度経度やお店の名前等
┃
┃
┃
┣━PlacePhotoの配列 お店の写真等の画像
┃
┃
┃
┗━PlaceReviewの配列 レビューコメントのリスト
┃
┃
┗━PlaceAspectRatingの配列
調べたAPI
google.maps.places.PlacesService- nearbySearch
- radarSearch
- textSearch
- getDetails
アイコンの表示
PlacesServiceで検索しただけでは、地図上にアイコン等は表示されません。アイコン等は自前で表示する必要があります。
アイコンの情報(url)は、PlaceResultの中に格納されているものを使用することができます。
以下のようなコードで表示できます。
var request = { ...検索条件を設定 }; placesService.nearbySearch(request, function(results, status) { if (status == google.maps.places.PlacesServiceStatus.OK) { for (var i = 0; i < results.length; i++) { var markerOption = { map: map, position: result[i].geometry.location, }; if (placeResult.icon != null) { var iconUrl = placeResult.icon; var icon = { url: iconUrl, scaledSize: new google.maps.Size(20, 20) }; markerOption.icon = icon; } else { var icon = { url: 'img/radar01.png', }; markerOption.icon = icon; } var marker = new google.maps.Marker(markerOption); } } };raderSearchのPlaceResultの中には、アイコン情報は格納されていないようなので、別途アイコンを用意しました。
nearBySearch
API呼び出し例
var request = { location: location, }; request.query = 'xxx'; ... その他、TextSearchRequest パラメータ設定 placesService.nearBySearch(request, function(results, status) { }
PlaceSearchRequestの設定に関して
Properties | コメント等 |
---|---|
bounds |
|
keyword | |
location | |
maxPriceLevel | |
minPriceLevel | |
name | |
openNow | |
radius |
|
rankBy | |
types |
PlaceResultのプロパティの取得状況
Properties | 取得の有無 |
---|---|
address_components | |
aspects | |
formatted_address | |
formatted_phone_number | |
geometry | あり |
html_attributions | |
icon | あり |
international_phone_number | あり |
name | あり |
permanently_closed | |
photos | あり |
place_id | あり |
price_level | あり |
rating | あり |
reviews | |
types | あり |
url | |
vicinity | |
website |
radarSearch
API呼び出し例
var request = { location: location, }; request.query = 'xxx'; ... その他、TextSearchRequest パラメータ設定 placesService.radarSearch(request, function(results, status) { }
RadarSearchRequestの設定に関して
Properties | コメント等 |
---|---|
bounds | boudsで指定した範囲内で検査が行われていました。 |
keyword | |
location | |
name | 指定した文字列で検索結果を絞り込んでいるようです。 |
radius | |
types |
PlaceResultのプロパティの取得状況
位置(geometry)とplace_id程度しか取得できません。Properties | 取得の有無 |
---|---|
address_components | |
aspects | |
formatted_address | |
formatted_phone_number | |
geometry | あり |
html_attributions | |
icon | |
international_phone_number | |
name | |
permanently_closed | |
photos | |
place_id | あり |
price_level | |
rating | |
reviews | |
types | |
url | |
vicinity | |
website |
textSearch
API呼び出し例
var request = { location: location, }; request.query = 'xxx'; ... その他、TextSearchRequest パラメータ設定 placesService.textSearch(request, function(results, status) { }
TextSearchRequestの設定に関して
Properties | コメント等 |
---|---|
bounds |
|
location | |
query |
|
radius |
|
types |
PlaceResultのプロパティの取得状況
nearBySearchと同じようです。getDetails
API呼び出し例
var request = { placeId: PlaceResultのplace_id等 }; placesService.getDetails(placeResult, function(detailResult, status) { }
PlaceDetailsRequestの設定に関して
Properties | コメント等 |
---|---|
placeId |
|
PlaceResultのプロパティの取得状況
getDetails では、ほとんどの項目に情報が格納されていました。登録してあれば、PlaceResultのすべてのプロパティが取得できるようです。
Properties | 内容 |
---|---|
address_components |
住所が、要素毎に分割されて配列として格納されています。 郵便番号、日本、東京都、渋谷区、... 等です。 |
aspects | |
formatted_address | 「日本, 〒xxx-xxxx 東京都渋谷区...」のような形式で住所が入っています。 |
formatted_phone_number | 電話番号です。 |
geometry | 位置(緯度、経度) |
html_attributions | |
icon | type毎のiconのurlが入っています。 |
international_phone_number | 電話番号です。+81 3-xxxx-xxxx の形式です。 |
name | 建物やお店の名前です。 |
permanently_closed | 閉店しているかという情報らしいです。 |
photos | 何枚か、写真が格納されています。 |
place_id | getDetails で使用する place_id が格納されています。 |
price_level | |
rating | |
reviews | |
types | nesrBySearchの対象となる typeが複数格納されています。 |
url | google+ のurl |
vicinity | 住所 |
website | web site の url |