[VC2005] 匿名メソッド実装によるスクリーンセーバー雛形
◆概要
この資料は、Microsoft(R) Visual C# 2005における 匿名メソッド実装によるスクリーンセーバー雛形について記述しています。
匿名メソッドを利用して、簡単にスクリーンセーバーを作るための雛形です。 この例では、ただ、画面を真っ暗にするだけです。 プレビューモード、オプションモードには対応していません。
◆1.新しいプロジェクトの作成新しくプロジェクトを作成します。ここでは、プロジェクト名に「ScreenSaverTemplate」としています。
![[新しいプロジェクト]ダイアログボックス](img/40-1.png)
◆2.Form1を削除する
プロジェクトが開かれたら、ソリューションエクスプローラでForm1を右クリックし、表示されたコンテキストメニューで[削除]0をクリックし、Form1を削除します。'Form1.cs'は完全に削除されます。というメッセージが表示されたら、[OK]ボタンをクリックします。
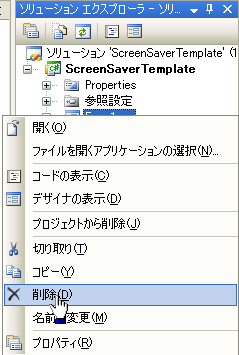
ソリューションエクスプローラから削除を選ぶ
◆3.フォームクラスを定義する
ソリューションエクスプローラでProgram.csをダブルクリックし、Program.csを開きます。
表示されたら、次のようにfrmMainクラスを定義します。
匿名メソッドを実装することによって、フォームがクリックされたり、マウスがクリックされるとフォームを閉じるようにしています。
class frmMain : Form { //マウスの位置を代入するための変数 private Point MouseXY; public frmMain() { //フォームを最大化 this.WindowState = FormWindowState.Maximized; this.BackColor = Color.Black; //ボーダースタイル無し this.FormBorderStyle = FormBorderStyle.None; this.TopMost = true; //最前面に表示 Cursor.Hide();//マウスカーソル非表示 this.Show(); // 匿名メソッドによるイベントハンドラ実装(パラメータ指定) MouseClick += delegate(object sender, MouseEventArgs e) { this.Close(); }; KeyDown += delegate(object sender, KeyEventArgs e) { this.Close(); }; MouseMove += delegate(object sender, MouseEventArgs e) { if (!MouseXY.IsEmpty) { if (MouseXY != new Point(e.X, e.Y)) Close(); if (e.Clicks > 0) Close(); } MouseXY = new Point(e.X, e.Y); }; } } |
▼ページトップへ
◆4.エントリーポイントの修正
static void Main()のApplication.Run(new Form1());のところを次のように書き換えます。
static class Program { /// <summary> /// アプリケーションのメイン エントリ ポイントです。 /// </summary> [STAThread] static void Main(string[] args) { Application.EnableVisualStyles(); Application.SetCompatibleTextRenderingDefault(false); args = Environment.CommandLine.Split(' '); //Length プロパティを調べて //コマンドライン引数があるかどうかを確認します if (args.Length != 0) { if (args[1].ToLower().StartsWith("/c")) { //設定モードのとき、通常はオプションダイアログを表示 //ここではノンサポート Application.Exit(); } else if (args[1].ToLower().StartsWith("/p")) { //プレビューモード //ここではノンサポート Application.Exit(); } else if (args[1].ToLower().StartsWith("/s")) { Application.Run(new frmMain()); } else {//コマンドライン引数がないとき Application.Run(new frmMain()); } } } } |
▼ページトップへ
◆全コード
using System; using System.Collections.Generic; using System.Windows.Forms; using System.Drawing; namespace ScreensaverTemplate1 { static class Program { /// <summary> /// アプリケーションのメイン エントリ ポイントです。 /// </summary> [STAThread] static void Main(string[] args) { Application.EnableVisualStyles(); Application.SetCompatibleTextRenderingDefault(false); args = Environment.CommandLine.Split(' '); //Length プロパティを調べて //コマンドライン引数があるかどうかを確認します if (args.Length != 0) { if (args[1].ToLower().StartsWith("/c")) { //設定モードのとき、通常はオプションダイアログを表示 //ここではノンサポート Application.Exit(); } else if (args[1].ToLower().StartsWith("/p")) { //プレビューモード //ここではノンサポート Application.Exit(); } else if (args[1].ToLower().StartsWith("/s")) { Application.Run(new frmMain()); } else {//コマンドライン引数がないとき Application.Run(new frmMain()); } } } } class frmMain : Form { //マウスの位置を代入するための変数 private Point MouseXY; public frmMain() { //フォームを最大化 this.WindowState = FormWindowState.Maximized; this.BackColor = Color.Black; //ボーダースタイル無し this.FormBorderStyle = FormBorderStyle.None; this.TopMost = true; //最前面に表示 Cursor.Hide();//マウスカーソル非表示 this.Show(); // 匿名メソッドによるイベントハンドラ実装(パラメータ指定) MouseClick += delegate(object sender, MouseEventArgs e) { this.Close(); }; KeyDown += delegate(object sender, KeyEventArgs e) { this.Close(); }; MouseMove += delegate(object sender, MouseEventArgs e) { if (!MouseXY.IsEmpty) { if (MouseXY != new Point(e.X, e.Y)) Close(); if (e.Clicks > 0) Close(); } MouseXY = new Point(e.X, e.Y); }; } } } |
▼ページトップへ